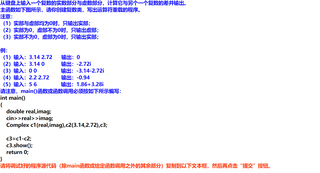
从键盘上输入一个复数的实数部分与虚数部分,计算它与另个一个复数的差并输出。
主函数如下图所示,请你创建复数类,写出运算符重载的程序。
注意:
(1)实部与虚部均为0时,只输出实部;
(2)实部为0,虚部不为0时,只输出虚部;
(3)实部不为0,虚部为0时,只输出实部;
#include <iostream>
using namespace std;
class Complex {
public:
Complex(double a = 0, double b = 0);
Complex operator-(const Complex& c);
friend ostream& operator<<(ostream& os, const Complex& c);
void show();
private:
double real;
double imag;
};
Complex::Complex(double a, double b) : real(a), imag(b) {}
Complex Complex::operator-(const Complex& c) {
return Complex(real - c.real, imag - c.imag);
}
/*
(1)实部与虚部均为0时,只输出实部;
(2)实部为0,虚部不为0时,只输出虚部;
(3)实部不为0,虚部为0时,只输出实部;
*/
ostream& operator<<(ostream& os, const Complex& c) {
if (c.imag == 0) {
os << c.real;
} else if (c.real == 0) {
os << c.imag << "i";
} else {
os << c.real << '+' << c.imag << "i";
}
return os;
}
void Complex::show() {
cout<<*this<<endl;
}
int main() {
double real, imag;
cin>>real>>imag;
Complex c1(real,imag),c2(3.14,2.72),c3;
c3 = c1 - c2;
c3.show();
return 0;
}
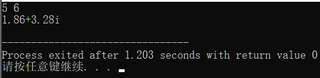
#include <iostream>
#include <cmath>
using namespace std;
class Complex
{
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
Complex operator-(const Complex& c) const {
return Complex(real - c.real, imag - c.imag);
}
void show() const {
if (real == 0 && imag == 0) {
cout << "0" << endl;
} else if (real == 0) {
cout << imag << "i" << endl;
} else if (imag == 0) {
cout << real << endl;
} else {
if (imag < 0)
cout << real << imag << "i" << endl;
else
cout << real << "+" << imag << "i" << endl;
}
}
private:
double real;
double imag;
};