import numpy as np
from matplotlib import pylab as pl
# Defining training data
x = np.array([1, 3, 2, 1, 3])
y = np.array([14, 24, 18, 17, 27])
# The regression equation takes the function
def fit(x, y):
if len(x) != len(y):
return
numerator = 0.0
denominator = 0.0
x_mean = np.mean(x)
y_mean = np.mean(y)
for i in range(len(x)):
numerator += (x[i] - x_mean) * (y[i] - y_mean)
denominator += np.square((x[i] - x_mean))
print('numerator:', numerator, 'denominator:', denominator)
b0 = numerator / denominator
b1 = y_mean - b0 * x_mean
return b0, b1
# Define prediction function
def predit(x, b0, b1):
return b0 * x + b1
# Find the regression equation
b0, b1 = fit(x, y)
print('Line is:y = %2.0fx + %2.0f' % (b0, b1))
# prediction
x_test = np.array([0.5, 1.5, 2.5, 3, 4])
y_test = np.zeros((1, len(x_test)))
for i in range(len(x_test)):
y_test[0][i] = predit(x_test[i], b0, b1)
# Drawing figure
xx = np.linspace(0, 5)
yy = b0 * xx + b1
pl.plot(xx, yy, 'k-')
pl.scatter(x, y, cmap=pl.cm.Paired)
pl.scatter(x_test, y_test[0], cmap=pl.cm.Paired)
pl.show()
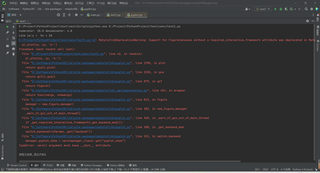