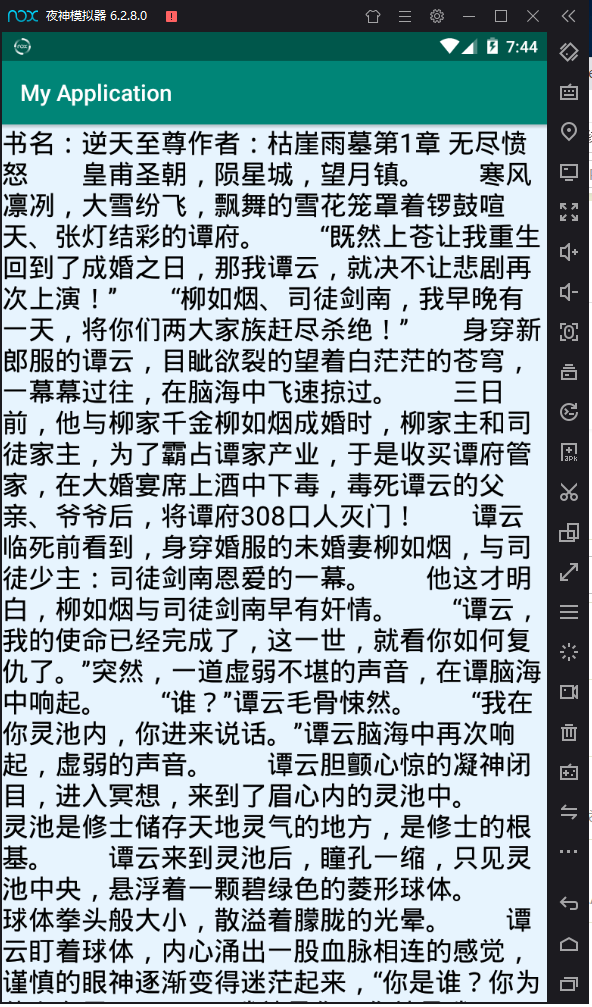
package com.example.myapplication;
import android.content.Intent;
import android.os.Handler;
import android.os.Message;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.text.method.ScrollingMovementMethod;
import android.widget.TextView;
import android.widget.Toast;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLConnection;
public class BookContent extends AppCompatActivity {
private TextView tv_con;
private String textPath = "";//txt文件网址
//private String content = new String(); //txt文件内容
private static final int CHANGE_CONTENT = 1;
private static final int ERROR = 2;
//主线程消息处理
private Handler handler = new Handler(){
public void handleMessage(Message msg){
if (msg.what == CHANGE_CONTENT) {
String content = (String)msg.obj;
tv_con.setText(content);
}else if(msg.what == ERROR){
Toast.makeText(BookContent.this,"显示文本内容错误!",Toast.LENGTH_LONG).show();
}
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_book_content);
Intent intent = getIntent();
textPath = intent.getStringExtra("textPath");
tv_con = (TextView) findViewById(R.id.tv_con);
getContentInfo();
tv_con.setMovementMethod(ScrollingMovementMethod.getInstance());
}
public void getContentInfo(){
//在子线程中获取服务器的数据
Thread thread = new Thread(){
HttpURLConnection conn;
@Override
public void run() {
//1:确定地址
try {
URL url = new URL(textPath);
//建立连接
conn = (HttpURLConnection) url.openConnection();
//设置请求方式
conn.setRequestMethod("GET");
//设置请求超时时间
conn.setConnectTimeout(500);
//设置读取超时时间
conn.setReadTimeout(500);
//判断是否获取成功
if(conn.getResponseCode() == 200) {
//获得输入流
InputStreamReader isReader = new InputStreamReader(conn.getInputStream(),"utf-8");
BufferedReader buffer = new BufferedReader(isReader);
String line = null;
StringBuffer sb = new StringBuffer();
while ((line = buffer.readLine()) != null) {
sb.append(line);
}
//将更改界面的消息发给主线程
Message msg = new Message();
msg.what = CHANGE_CONTENT;
msg.obj = sb.toString();//将文本信息传给obj
handler.sendMessage(msg);
} else{
Message msg = new Message();
msg.what = ERROR;
handler.sendMessage(msg);
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
Message msg = new Message();
msg.what = ERROR;
handler.sendMessage(msg);
}
//关闭连接
conn.disconnect();
}
};
//启动线程
thread.start();
}
public String getText(String textPath){
int HttpResult;
StringBuffer sb = new StringBuffer();
String line = null;
BufferedReader buffer = null;
try {
URL url = new URL(textPath);
URLConnection conn = url.openConnection();
conn.connect();
HttpURLConnection httpconn = (HttpURLConnection)conn;
HttpResult = httpconn.getResponseCode();
if (HttpResult != HttpURLConnection.HTTP_OK){
Toast.makeText(this, "解析失败", Toast.LENGTH_SHORT).show();
}else {
InputStreamReader isReader = new InputStreamReader(conn.getInputStream(),"utf-8");
buffer = new BufferedReader(isReader);
while ((line = buffer.readLine()) != null) {
if (line == "第"+"章"){
sb.append("\n"+line+"\n");
}
sb.append(line);
}
}
return sb.toString();
}catch (Exception e){
e.printStackTrace();
return sb.toString();
}
}
}